2 Union vs. Enum in Rust: Understanding Memory Layout
union MyUnion {
a: i32, //4 bytes
b: f64, //8 bytes
c: (i32, i32), //8 bytes
}
mem::size_of::<MyUnion>() -> 8 bytes
The size is based on the largest member.
enum WebEvent {
// An `enum` variant may either be `unit-like`,
PageLoad, // 1 byte
PageUnload, // 1 byte
// like tuple structs,
KeyPress(char), // 1 byte
// or c-like structures.
Click { x: i64, y: i64 }, // 16 bytes
}
mem::size_of::<MyUnion>() -> 24 bytes
The size of determined by the size of the largest vaiant plus the size of the additional identifier(tag) and then aligned to the required memory alignment.
enum WebEvent {
// An `enum` variant may either be `unit-like`,
PageLoad, // 1 byte
PageUnload, // 1 byte
// like tuple structs,
KeyPress(char), // 1 byte
Paste(String), // 24 bytes
// or c-like structures.
Click { x: i64, y: i64 }, // 16 bytes
}
The size of the WebEvent should be:
24bytes + 1byte(tag) = 25bytes.
After applying 8-byte alignment:
24bytes + 1byte(tag) = 25bytes = 32bytes
BUT:
mem::size_of::<WebEvent>() -> 24 bytes
Why?
let pressed = WebEvent::KeyPress('x');
// `to_owned()` creates an owned `String` from a string slice.
let pasted = WebEvent::Paste("my text".to_owned());
let click = WebEvent::Click { x: 20, y: 80 };
let load = WebEvent::PageLoad;
let unload = WebEvent::PageUnload;
load


unload

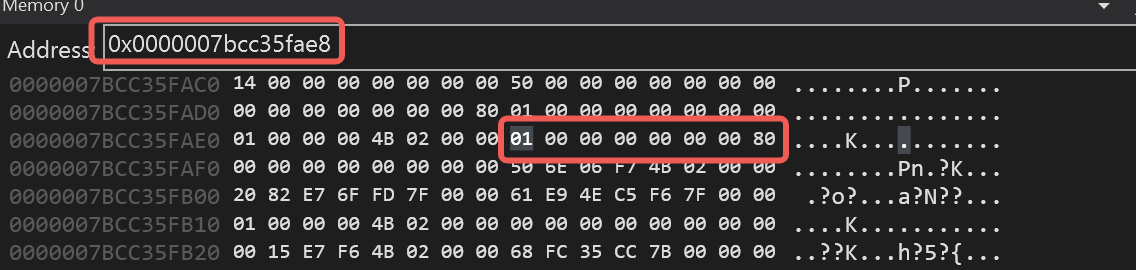
pressed

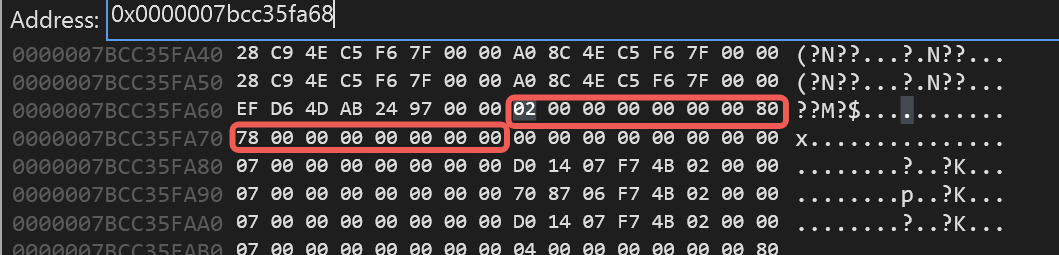
click
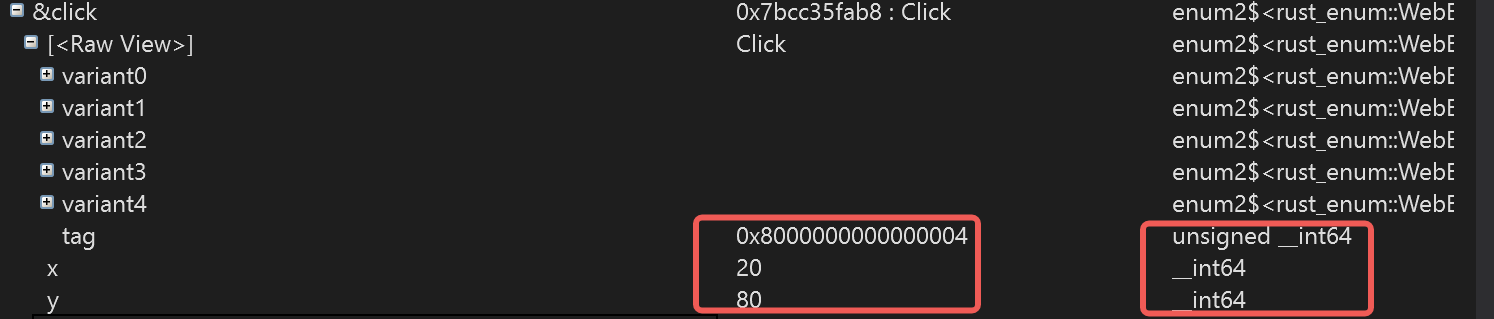
pasted

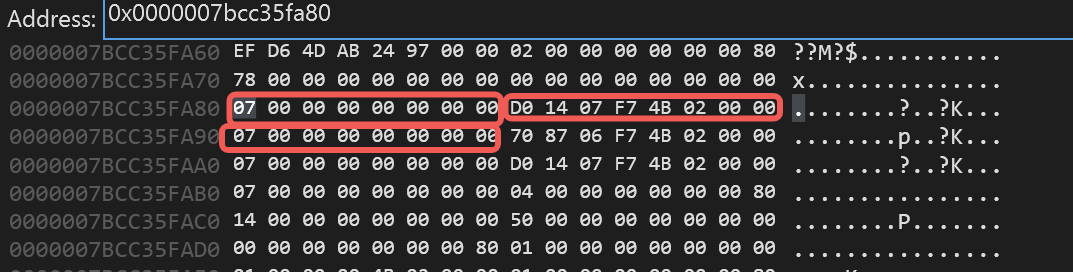

Enum
The size of an enum is defined by the size of its largest variant, along with an additional identifier that tracks which variant is currently active.
Provides type safety and pattern matching. Rust checks at compile time that all possible variants are handled, ensuring that you cover every case.
Union
The size of a union is only determined by its largest member, as all members share the same memory space.
Is considered unsafe; accessing union members requires being within an unsafe block.
Rust does not check if you are accessing the member in the correct context.
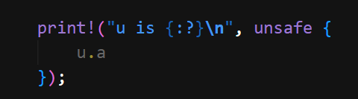
The member types of a union should not include destructors (such as the Drop trait).
This means that if a struct holds resources (such as heap-allocated memory), it can lead to memory leaks or undefined behavior.
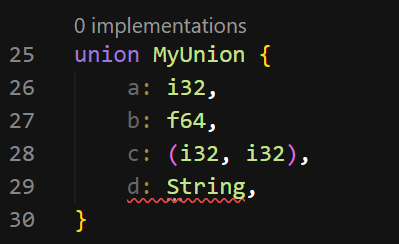
